Have you ever wondered how websites and applications ensure the accuracy of the phone numbers you enter during registration or contact forms? Phone number validation is a crucial aspect of web development that helps you check phone number accuracy. Moreover, it prevents errors that could lead to communication issues. In this blog, we will delve into creating a phone number checker using HTML and JavaScript. Furthermore, it sheds light on why it is an essential practice for developers.
Validating phone numbers goes beyond merely checking for digits; it involves verifying the structure, country codes, and number types. Understanding the significance of phone number validation will help you create more reliable applications. Throughout this blog, we will explore the concept of a phone number checker, its importance in data quality, and its benefits to end-users.
Moreover, we will introduce you to Numverify, a powerful API that simplifies the process of phone number validation. Let’s begin.
Table of Contents
What Is Phone Number Validation?
Phone number validation verifies the accuracy of user phone numbers in web forms or applications. It ensures that the provided phone numbers are in the correct format and adhere to specific rules based on the country or region. By validating phone numbers, developers can prevent errors and improve data quality. Ultimately enhancing communication efficiency.
Phone number validation is essential in reducing the possibility of incorrect or fake numbers. Hence, ensuring that messages and calls reach the intended recipients without disruptions or delivery failures.
Why Do We Need to Validate Phone Numbers?
Phone number validation is a crucial practice in web development for several reasons.
Firstly, it ensures data accuracy and reliability by verifying the format and structure of phone numbers. Validated phone numbers contribute to better data quality and minimize the risk of incorrect or incomplete information.
Secondly, phone number validation enhances user experience. Preventing the submission of fake or erroneous numbers reduces frustration and ensures that users are reachable through phone calls without any issues.
Additionally, validated phone numbers improve communication efficiency. Businesses and organizations can rely on accurate contact information to send important updates, notifications, or marketing messages. Hence, fostering effective communication with their target audience.
Lastly, phone number validation aids in fraud prevention. By filtering out fake or invalid numbers, it reduces the risk of fraud and protects users and businesses from potential scams or malicious activities.
What Are the Features of a Best Phone Number Validator?
A reliable phone number validator has essential features to ensure accurate and efficient validation.
Firstly, it should handle international phone numbers. Furthermore, it accommodates various country codes and formats. The best validators, offering comprehensive verification, can detect and differentiate between mobile, landline, and other number types.
An ideal validator should provide real-time feedback during input, immediately alerting users of errors or discrepancies.
Moreover, the validator must have built-in error handling mechanisms, gracefully handling unexpected inputs or API failures.
It should be highly customizable. Hence allowing developers to tailor the validation process to their specific needs.
The best validators prioritize security. Hence ensuring that user data remains confidential and secure during validation.
Lastly, seamless integration capabilities with popular frameworks and programming languages make the validator more developer-friendly.
What Is Numverify?
NumVerify is a valuable resource for validating the authenticity of any phone number. The verification process can be conducted independently on the back end or seamlessly integrated into websites or applications through the NumVerify API. Hence, allowing users to verify their numbers effortlessly.
Operating on a RESTful JSON API, NumVerify covers phone numbers from 232 countries worldwide, encompassing domestic and international numbers. Each inquiry involves cross-referencing the number against up-to-date numbering plan databases.
NumVerify phone verification tool offers a free trial with up to 100 demo API requests. For more extensive usage, users can opt for Basic, Professional, or Enterprise plans at affordable monthly prices, ranging from $9.99 to $99.99. Choosing to upgrade after experiencing the service’s efficiency is highly recommended.
How Does Numverify Work?
Upon registration with NumVerify, you’ll receive an exclusive API access key. The API key serves as authentication for your account’s API requests. With this access key and the phone number, you can seamlessly utilize NumVerify services.
The process begins on your NumVerify dashboard, where a user-friendly validation form is accessible alongside your API access key.
To perform a reverse search on any phone number, input it with the corresponding country code and click the Run button.
Subsequently, the JSON results will appear in a new browser window, presenting comprehensive details about the queried phone number straightforwardly and concisely.
This convenient landing page offers all the essential information for accurate phone number validation.
A detailed Numverify response should look like the below:
{
"valid": true,
"number": "14158586273",
"local_format": "4158586273",
"international_format": "+14158586273",
"country_prefix": "+1",
"country_code": "US",
"country_name": "United States of America",
"location": "Novato",
"carrier": "AT&T Mobility LLC",
"line_type": "mobile"
}
Note that when your API request fails, this is the error that you may get through Numverify.
{
"success": false,
"error": {
"code": 210,
"type": "no_phone_number_provided",
"info": "Please specify a phone number. [Example: 14158586273]"
}
}
Now that you have learned about Numverify, you must know how to implement it. I have provided a step-by-step tutorial below.
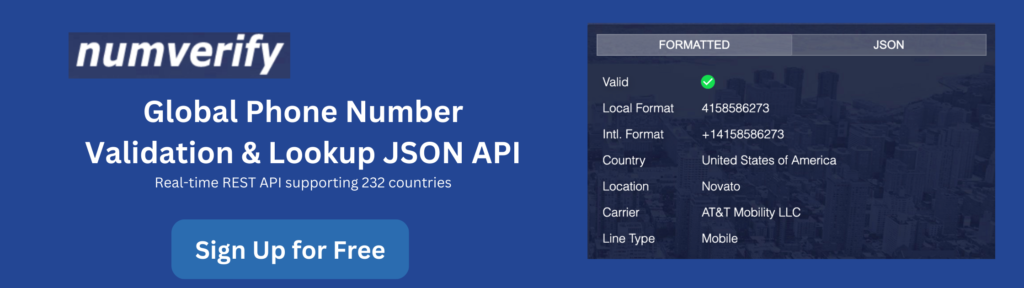
How to Validate Numbers Using Numverify?
Here are the simple steps that you should follow.
Before following the steps below, ensure you have registered yourself on Numverify. It will help you get the Numverify API key. It will also help you assess other endpoints available at the Numverify website.
Step 1: Set Up the HTML Structure
Start by creating an HTML file and set up the basic structure. Include the necessary `<html>`, `<head>`, and `<body>` tags. Inside the `<head>` tag, add a `<title>` to give your page a relevant title. Also, link an external CSS file (optional) to style the form later.
<!DOCTYPE html>
<html>
<head>
<title>Registration Form with Phone Number Validation</title>
<link rel="stylesheet" href="styles.css"> <!-- Optional: External CSS file for styling -->
</head>
<body>
Step 2: Create the Registration Form
Next, add the registration form inside the `<body>` section. Create labels and input fields for name, email, and phone number. Use appropriate `type` attributes for email and phone number inputs to enable validation. Add a button to submit the form and call the `validatePhoneNumber()` function on click.
<h2>Registration Form</h2>
<form id="registrationForm">
<label for="name">Name:</label>
<input type="text" id="name" required><br><br>
<label for="email">Email:</label>
<input type="email" id="email" required><br><br>
<label for="phone">Phone Number:</label>
<input type="tel" id="phone" required><br><br>
<button type="button" onclick="validatePhoneNumber()">Submit</button>
</form>
Step 3: Implement Phone Number Validation Function
In the `<script>` section, define the `validatePhoneNumber()` function. Inside the function, get the phone number input value using `getElementById()` and store it in a variable. Replace `’YOUR_NUMVERIFY_API_KEY’` with your actual Numverify API key to access the API.
<script>
function validatePhoneNumber() {
const phoneNumberInput = document.getElementById("phone");
const phoneNumber = phoneNumberInput.value;
// Replace 'YOUR_NUMVERIFY_API_KEY' with your actual API key
const apiKey = "YOUR_NUMVERIFY_API_KEY";
const url = `http://apilayer.net/api/validate?access_key=${apiKey}&number=${encodeURIComponent(phoneNumber)}`;
// Making the API request and handling response
fetch(url)
.then(response => response.json())
.then(data => {
if (data.valid) {
alert("Phone number is valid!");
} else {
alert("Invalid phone number. Please check and try again.");
}
})
.catch(error => {
alert("Phone number validation failed. Please try again later.");
console.error(error);
});
}
</script>
```
Styling Our Web Form
Step 1: Create an External CSS File
Start by creating an external CSS file (e.g., `styles.css`) and save it in the same directory as your HTML file. This will keep your CSS code organized and separate from your HTML code.
Step 2: Set Font and Margin for Body
In the `styles.css` file, start by setting the font family to “Arial, sans-serif” and adding a 20px margin to the body to create spacing around the form.
body {
font-family: Arial, sans-serif;
margin: 20px;
}
Step 3: Center the Heading
Next, style the `h2` element to center the heading and add a 20px bottom margin for some spacing between the heading and the form.
h2 {
text-align: center;
margin-bottom: 20px;
}
Step 4: Style the Form Container
For the form container, apply a maximum width of 400px and center it using `margin: 0 auto`. Add padding, a 1px solid border with a light gray color, and a subtle box shadow to create a card-like appearance.
form {
max-width: 400px;
margin: 0 auto;
padding: 20px;
border: 1px solid #ccc;
border-radius: 5px;
background-color: #fff;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.1);
}
Step 5: Style Labels and Input Fields
Style the `label` elements to display as blocks with a bold font weight and add a 5px bottom margin for some spacing between labels and input fields. Style the `input` fields with a 100% width, 10px padding, 15px bottom margin, a 1px solid border with a light gray color, and a 5px border-radius to create rounded corners.
label {
display: block;
font-weight: bold;
margin-bottom: 5px;
}
input[type="text"],
input[type="email"],
input[type="tel"] {
width: 100%;
padding: 10px;
margin-bottom: 15px;
border: 1px solid #ccc;
border-radius: 5px;
}
Step 6: Style the Submit Button
Style the `button` element with a green background color (#4CAF50) and white text color. Add 10px padding on the top and bottom and 20px padding on the left and right. Remove the default button border and add a 5px border radius for rounded corners. Change the cursor to a pointer on hover for a better user experience.
button {
background-color: #4CAF50;
color: white;
padding: 10px 20px;
border: none;
border-radius: 5px;
cursor: pointer;
width: 100%;
}
button:hover {
background-color: #45a049;
}
Step 7: Link the CSS File to the HTML
Finally, link the `styles.css` file to your HTML file. Inside the `<head>` section, add the following line to connect the CSS file to your HTML:
<link rel="stylesheet" href="styles.css">
That’s it! With these CSS styles, your registration form will be attractive and user-friendly. Hence making it visually appealing for your users.
Now, let’s move to our next steps for web form.
Step 4: Test the Phone Number Validation
Save the file and open it in a web browser. Enter some sample data into the registration form, including a valid or invalid phone number. When you click the “Submit” button, the `validatePhoneNumber()` function will execute and make an API call to Numverify for phone number validation.
If the phone number is valid, you will see an alert saying, “Phone number is valid!” Otherwise, you will see an appropriate error message if the phone number is invalid or the API request fails.
When the number is invalid:
When the number is valid:
Congratulations! You have successfully created a registration form with phone number validation using HTML, JavaScript, and the Numverify API.
Conclusion
Phone number validation is a vital aspect of web development, ensuring the accuracy and reliability of user data. Developers can enhance user experience, prevent communication errors, and maintain data quality using HTML and JavaScript for the validation of phone numbers. Integrating the Numverify API offers a seamless and efficient solution. Hence providing real-time feedback on phone number validity, type, and origin.
With step-by-step guidance, we have explored the significance of phone number validation, its features, and the benefits it brings to web applications. This powerful tool allows developers to create more robust and user-friendly platforms. Hence fostering smoother communication and interactions.
FAQs
Can I Find Out Who a Phone Number Belongs To?
You can find out who a phone number belongs to using reverse phone lookup services or online directories.
Can You Google Search for a Phone Number?
You can perform a Google search for a phone number to find information or identify the caller.
How Can I Find a Phone Number for Free?
You can find a phone number for free through online directories, social media platforms, or search engines.
How Can I Trace Someone by Mobile Number?
Tracing someone by mobile number requires access to private databases, which are not publicly available. Seek legal assistance if needed.
Sign Up for Free at Numverify Today to get the phone numbers validated.
