Precise details about phone carriers are helpful in various scenarios, such as enhancing user satisfaction, targeted marketing, preventing fraud, and enhancing security measures. Knowing someone’s carrier information can also help with identity verification or validation. Thus, developers and businesses often integrate carrier information retrieval functionality into their apps. But what’s the best way to integrate this functionality into your app to find phone carrier details?
This guide will examine how to find phone carrier information using Python. For Python, we’ll show you two ways to find carrier by Phone number. We’ll go over both API-based solutions and regular expression methods. You can choose the most suitable approach for your project to integrate the mobile carrier lookup functionality.
When you finish reading this guide, you will have the ability to incorporate carrier information retrieval into your applications with ease. Let’s get started!
Table of Contents
What do I need to Find Phone Carrier Information?
Here are the key requirements for integrating phone carrier lookup functionality in your app:
Basic Understanding of Python
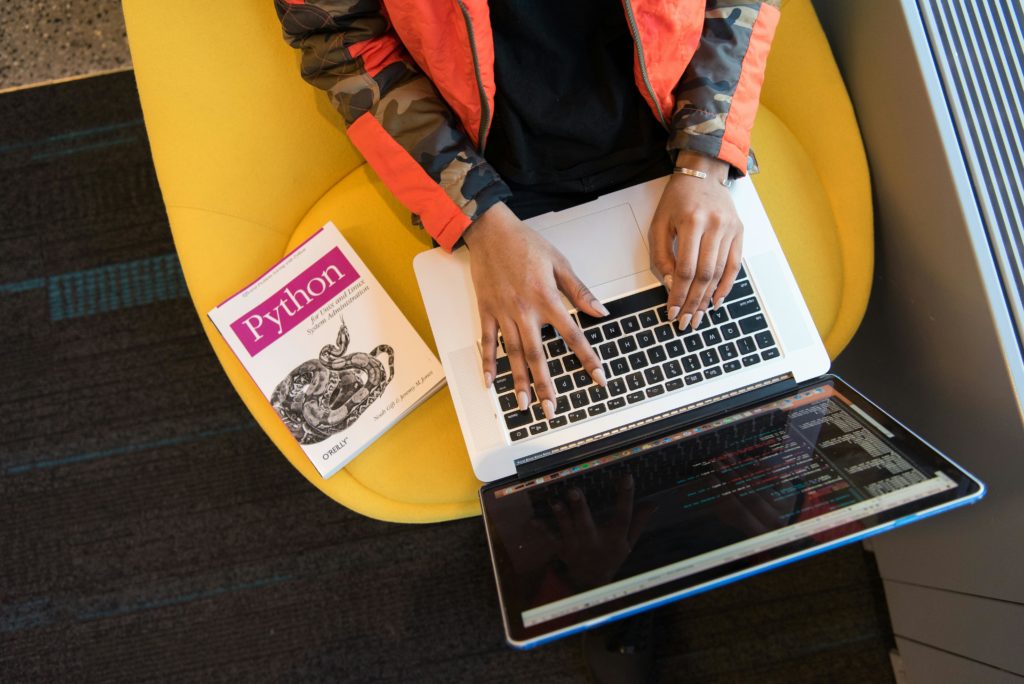
Although we’ll walk you through the required procedures, having a basic understanding of Python concepts would be beneficial. For instance, you can customize the code further according to your project’s requirements.
Functional Python Environment
To execute the Python code examples given in this guide, it is necessary to have a working Python environment. If you do not have Python installed on your computer, you can download and install it from the official Python website. It is advisable to use Python 3. x for better compatibility.
Basic terminal or command prompt usage
It is important to have proficiency in using the terminal or command prompt to execute commands. This involves tasks such as installing packages through pip or npm.
Numverify Carrier Lookup API Key
We’ll also need a carrier lookup API to retrieve carrier details/information. For this guide, we’ll use the numverify API, a global phone number validation and lookup API. We’ve chosen numverify because of various reasons:
- Numverify is widely recognized for its high accuracy in phone number validation, carrier detection and line type detection. Thus, it’s a dependable source to find phone carrier information, thanks to its comprehensive and reliable database.
- It supports both local and international phone numbers and offers global coverage. This makes numverify a great choice for projects with diverse user bases.
- Numverify provides real-time responses. This ensures that you always get the latest carrier details for the phone numbers you provide.
- Integrating Numverify into your Python or JavaScript projects is easy and hassle-free. The API has extensive documentation with clear usage instructions and code examples.
- Numverify is a developer-friendly API that caters to programmers of all skill levels.
- It offers the ability to use phone number lookup free of charge for up to 1000 times a month.
Note: You need to sign up and get the numverify API key to implement the code examples provided in this guide or to use numverify in any app/code.
How to Find Phone Carrier Information in Python?
There are two methods for finding phone carriers using Python. One method involves using the numverify API, whereas the other involves using regular expressions.
Using a Carrier Lookup API to Find Phone Carrier Information
If you’re looking for a dependable and advanced method to identify phone carrier information, then a Carrier Lookup API is the way to go. These APIs connect with substantial databases that contain information about phone numbers, making it easy to obtain precise carrier details.
Here are the key steps to integrate a carrier lookup API, such as numverify, into your Python application to find phone carrier information:
Sign Up and Get the API Key
To start, go to the numverify website and sign up for a user account. After registering, log in to your account and go to the API section. From there, you can generate an API key. The API key will serve as an authentication token, allowing you to access numverify’s features and endpoints.
Install Required Packages
We will utilize the requests library in Python to manage API responses and perform HTTP requests. If the library is not yet installed, open the terminal or command prompt and execute the command following command:
pip install requests
Writing Python Code
Next, we will write Python code that can interact with the phone carrier lookup tool/numverify API. We’ll develop a function called ‘get_carrier_info’ that will require the API key and a phone number as inputs. This function will create an API endpoint using the provided inputs, then send a GET request and extract the carrier information from the JSON response.
Here is the code:
import requests
def get_carrier_info(phone_number, api_key):
endpoint = f"http://apilayer.net/api/validate?access_key={api_key}&number={phone_number}"
response = requests.get(endpoint)
data = response.json()
return data.get("carrier", "")
Calling the API Function
Once you have defined the function, you can enter phone number and then display the corresponding carrier information:
api_key = 'YOUR_API_KEY'
phone_number = "+1234567890"
carrier_info = get_carrier_info(phone_number, api_key)
print("Carrier:", carrier_info)
Make sure to replace ‘YOUR_API_KEY’ with your actual API key.
After the search is complete, you will receive details about the carrier that is connected to the phone number you provided.
Here is the complete code:
import requests
def get_carrier_info(phone_number, api_key):
endpoint = f"http://apilayer.net/api/validate?access_key={api_key}&number={phone_number}"
response = requests.get(endpoint)
data = response.json()
return data.get("carrier", "")
api_key = 'YOUR_API_KEY'
phone_number = "+1234567890"
carrier_info = get_carrier_info(phone_number, api_key)
print("Carrier:", carrier_info)
Output:
Using Regular Expressions
Although Carrier Lookup APIs are very accurate, if you’re creating a simple app, you can use regular expressions to find phone carrier information in-house. Regular expressions are patterns that are used to match and manipulate strings. In this approach, we will investigate how to use regular expressions to carry out a numlookup.
Here are the key steps to find a carrier by phone number using regular expressions:
Writing Python Code
You can use regular expressions to find particular patterns within strings. To extract information that resembles a phone number carrier, we will develop a function named extract_carrier_info. This function will take a phone number as input and use a regular expression pattern to locate and extract relevant carrier information.
import re
def extract_carrier_info(phone_number):
carrier_pattern = re.compile(r"\b\d{3}-\d{3}-\d{4}\b") # Customize based on your country's phone number format
match = carrier_pattern.search(phone_number)
if match:
return "Sample Carrier" # Replace with actual carrier information
else:
return "Carrier information not found."
If you want to focus on a particular type of phone number format, you can modify the pattern in the carrier_pattern variable. Simply adjust it according to the phone number format used in your country.
Calling the Function
Once you have defined the function, you can input a phone number as a sample and then print out the extracted carrier information.
phone_number = "123-456-7890" # Replace with your phone number
carrier_info = extract_carrier_info(phone_number)
print("Carrier:", carrier_info)
If the phone number entered meets the designated format, you will be presented with details similar to those provided by the phone carrier. However, if the information is not found, you will receive a notification informing you of the same.
Here is the complete code:
import re
def extract_carrier_info(phone_number):
carrier_pattern = re.compile(r"\b\d{3}-\d{3}-\d{4}\b") # Customize based on your country's phone number format
match = carrier_pattern.search(phone_number)
if match:
return "Sample Carrier" # Replace with actual carrier information
else:
return "Carrier information not found."
phone_number = "123-456-7890" # Replace with your phone number
carrier_info = extract_carrier_info(phone_number)
print("Carrier:", carrier_info)
Output:
Conclusion
If you’re looking to integrate precise carrier data into your Python projects to enhance user satisfaction, streamline workflows, and enhance data validation, you can follow this guide. We’ve covered two methods to find phone carrier information in Python. The first method involves using a carrier lookup API. We’ve used the numverfiy API in our example as it’s known for its accurate database and quick response time. The second method involves using regular expressions in Python. This method is more suitable for simpler apps. For big projects, we recommend using the API method.
Head over to Numverify and create an account to obtain your API key today!
FAQs
How to find the carrier of a phone?
You can use an online carrier lookup service to find the carrier associated with a phone number. However, if you’re looking to integrate the carrier lookup functionality into your apps to find phone carrier information, you can use an accurate and reliable API like numverify.
How can I trace a phone number’s details?
You can use numverify to validate phone numbers. Numverify also supports carrier and line-type detection.
How does a phone carrier lookup work?
You need to enter the phone number, and the carrier lookup service will return the relevant information.
What is a carrier code in number?
It is the small code at the start of a number. It can be used to identify the network provider of that particular number.